You can very easily customise the appearance of all Pulsate SDK's UI so it fits your needs. You can change colors of all elements and the content of texts.
Pulsate SDK 4.7.0
Pulsate SDK 4.7.0 now uses a Web Feed, Custom Theming is no longer available on the App side. When creating campaigns in the Campaign Builder you can specify the color of the buttons and text in Feed Posts. We do plan to add more Custom Theming in future releases if there is demand for it.
In Apps can still have custom themed directly from the SDK using the Theme Configuration:
let manager = PULPulsateFactory.getDefaultInstance()
let config = PULThemeConfiguration()
config.bigInAppOneButtonColor = UIColor.red
config.bigInAppOneButtonTextColor = UIColor.white
manager?.applyThemeConfiguration(config)
Pulsate SDK 4.2.0
Pulsate SDK 4.2.0 allows you to store your configuration files locally. By default both colors and string jsons are stored in the podfile. Every podfile update will download a new pod and all configurations will be lost and you need to update / replace the jsons.
In Pulsate SDK 4.2.0 the Pulsate config for strings and colors can now be moved into your App files. Pod updates will not delete your local config. You first need to copy configurations from the pulsatebasic bundle and add them into the main app, then you need to pass the main app bundle id to manager.resourcebundleIdentifier. Example for app with identifier pulsate.universal.app - pulsateManager.resourceBundleIdentifier = "pulsate.universal.app", with this change the SDK will now search for resources in the main folder of the App.
Pulsate Configuration JSON
The pulsateConfiguration.json file can be found the PULPulsateBasic.bundle. The easiest way to find the file is just to type it's name into XCode's explorer.
If you want to use a custom color, just put a hexadecimal color in the element you want to change. If you want to color the navigation bar to yellow just put a hexadecimal number in the corresponding element "pulsate_top_nav_bar_background_color" : "#ffde17"
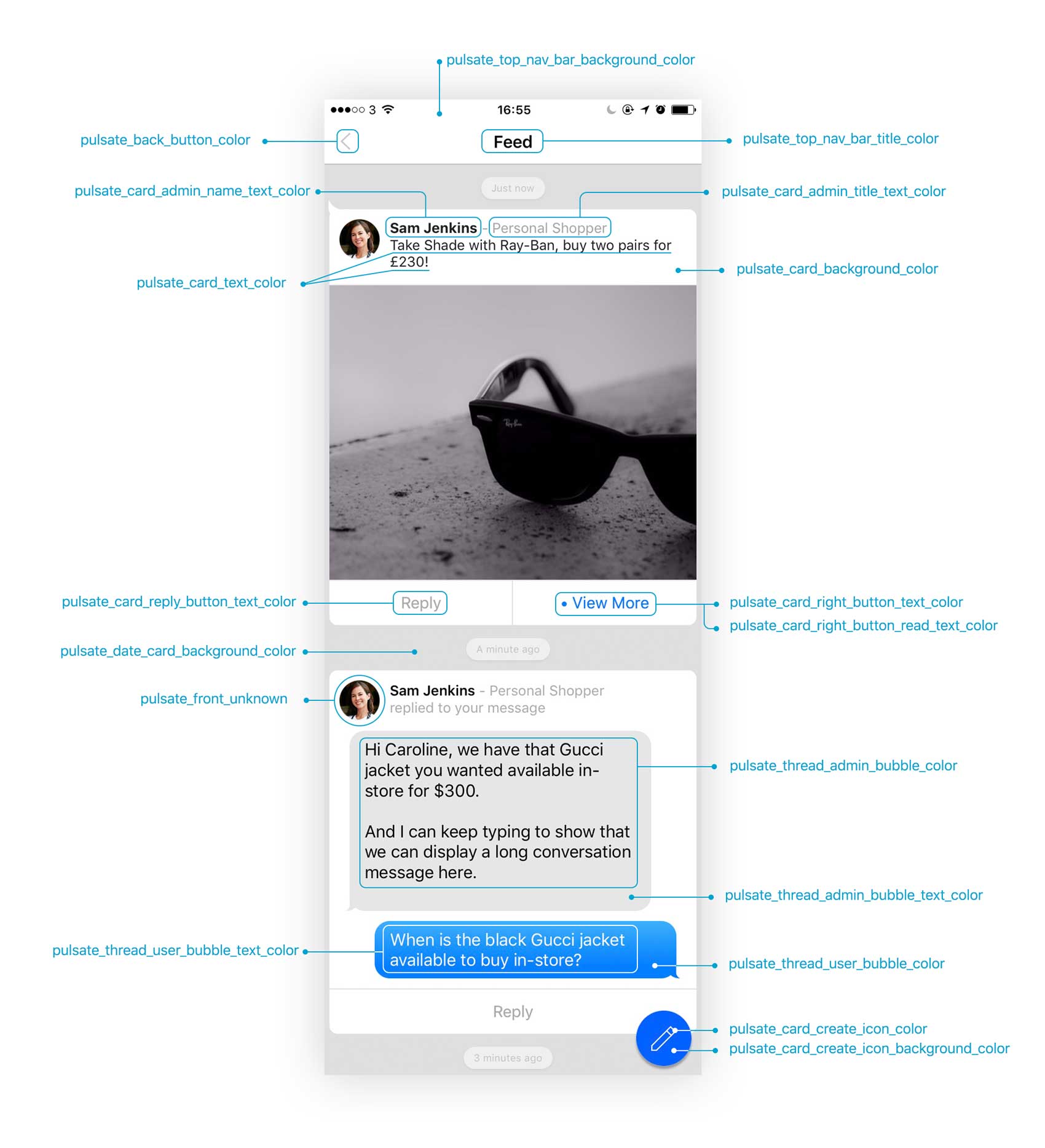
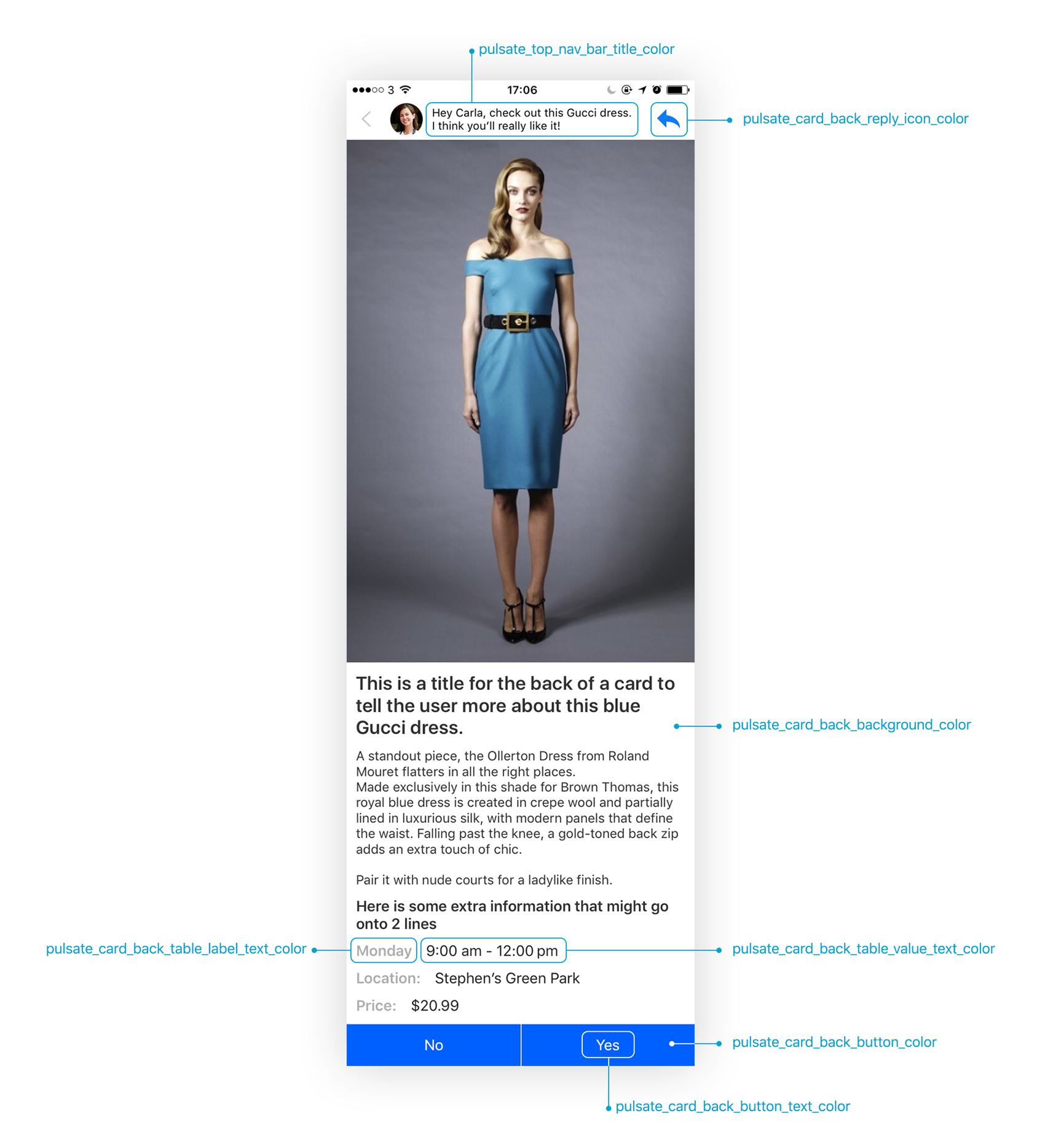
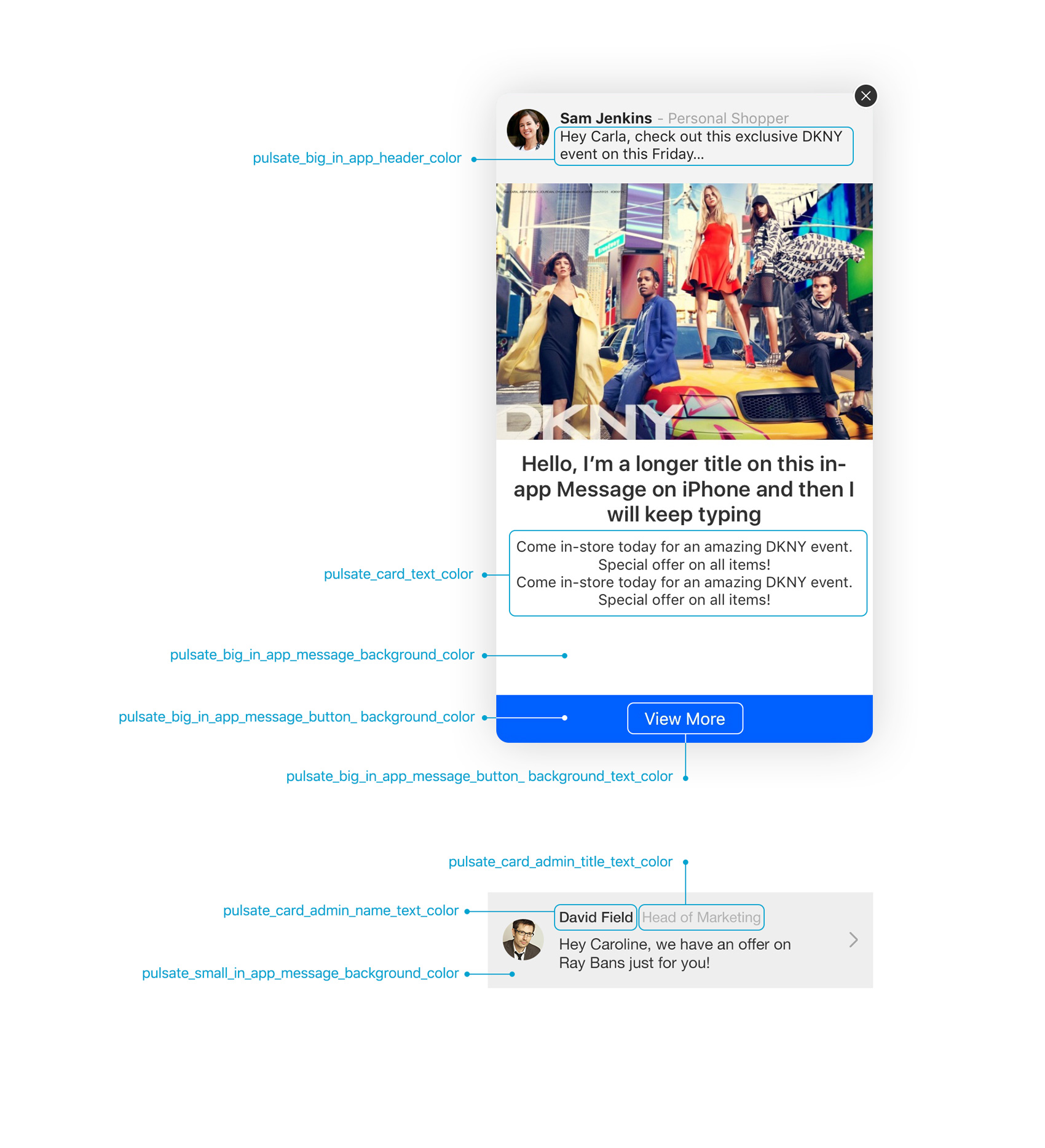
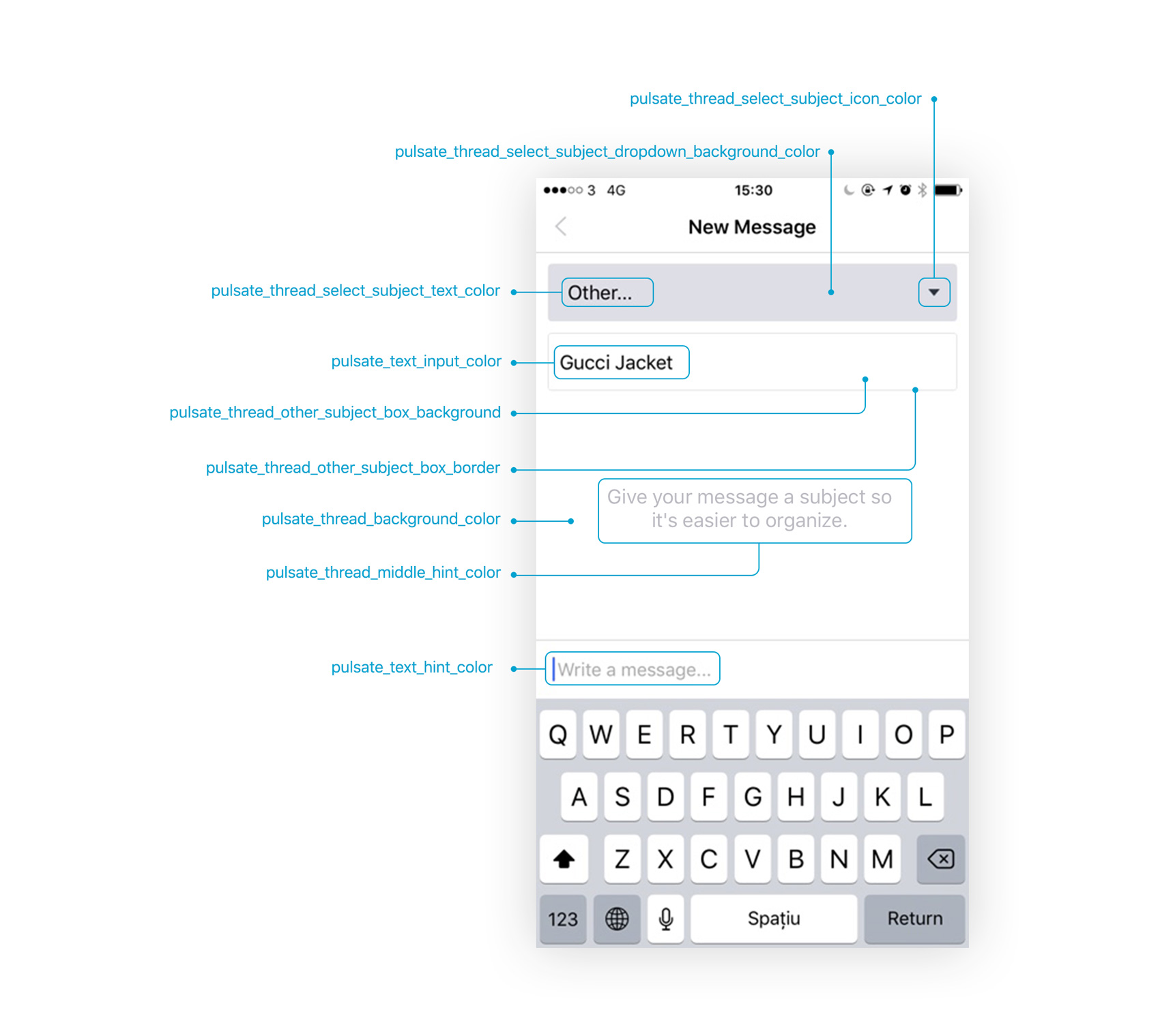
Pulsate Toolbar Customization
With the release of version 2.14.12 Developers can now add an additional button in the Pulsate Feed. The button will appear in the main Feed Toolbar in the right corner.
To add this button you need to call the new setInboxRightButton method and pass an UIBarButtonItem that you want to show. This should be done in the AppDelegate under didFinishLaunchingWithOptions.
UIButton* _helpButton = [UIButton buttonWithType:UIButtonTypeSystem];
[_helpButton setTitle:@"Help" forState:UIControlStateNormal];
[_helpButton sizeToFit];
[_helpButton addTarget:self action:@selector(helpPressed) forControlEvents:UIControlEventTouchUpInside];
UIBarButtonItem* helpItem = [[UIBarButtonItem alloc] initWithCustomView:_helpButton];
[pulsateManager setInboxRightButton:helpItem];
-(void) helpPressed {
}
let helpButton = UIBarButtonItem(
title: "Help",
style: .plain,
target: self,
action: #selector(helpPressed(sender:))
)
pulsateManager.setInboxRightButton(helpButton)
@objc func helpPressed(sender: UIBarButtonItem) {
}
User Avatar Customization
The user avatar by default is set to use the user initials when possible, when not available the user avatar will be an anonymous icon. With the release of version 2.14.12 Developers can now disable showing initials as the user avatar, this will cause the avatar to be the anonymous icon even if FirstName and LatName are set. This can be done by calling the new useInitialsForUserAvatar method. This should be done in the AppDelegate under didFinishLaunchingWithOptions.
[pulsateManager useInitialsForUserAvatar:NO];
pulsateManager.useInitials(forUserAvatar: false)
Font Customization
To change the fonts that are used in the Pulsate Inbox Developers can override the UIFont class and set default fonts for UITextField and UILabel in their AppDelegate.
Please create the following files in your project - UIFont+SystemFontOverride.h and UIFont+SystemFontOverride.m. The font we will use in this Demo is called "PartyLetPlain", change this font to any other you want to use.
// UIFont+SystemFontOverride.h
#import <UIKit/UIKit.h>
@interface UIFont (SystemFontOverride)
@end
// UIFont+SystemFontOverride.m
#import <objc/runtime.h>
#import "UIFont+SystemFontOverride.h"
NSString *const FORegularFontName = @"PartyLetPlain";
NSString *const FOBoldFontName = @"PartyLetPlain";
NSString *const FOItalicFontName = @"PartyLetPlain";
@implementation UIFont (SystemFontOverride)
#pragma clang diagnostic push
#pragma clang diagnostic ignored "-Wobjc-protocol-method-implementation"
+ (void)replaceClassSelector:(SEL)originalSelector withSelector:(SEL)modifiedSelector {
Method originalMethod = class_getClassMethod(self, originalSelector);
Method modifiedMethod = class_getClassMethod(self, modifiedSelector);
method_exchangeImplementations(originalMethod, modifiedMethod);
}
+ (void)replaceInstanceSelector:(SEL)originalSelector withSelector:(SEL)modifiedSelector {
Method originalDecoderMethod = class_getInstanceMethod(self, originalSelector);
Method modifiedDecoderMethod = class_getInstanceMethod(self, modifiedSelector);
method_exchangeImplementations(originalDecoderMethod, modifiedDecoderMethod);
}
+ (UIFont *)regularFontWithSize:(CGFloat)size
{
return [UIFont fontWithName:FORegularFontName size:size];
}
+ (UIFont *)boldFontWithSize:(CGFloat)size
{
return [UIFont fontWithName:FOBoldFontName size:size];
}
+ (UIFont *)italicFontOfSize:(CGFloat)fontSize
{
return [UIFont fontWithName:FOItalicFontName size:fontSize];
}
- (id)initCustomWithCoder:(NSCoder *)aDecoder {
BOOL result = [aDecoder containsValueForKey:@"UIFontDescriptor"];
if (result) {
UIFontDescriptor *descriptor = [aDecoder decodeObjectForKey:@"UIFontDescriptor"];
NSString *fontName;
if ([descriptor.fontAttributes[@"NSCTFontUIUsageAttribute"] isEqualToString:@"CTFontRegularUsage"]) {
fontName = FORegularFontName;
}
else if ([descriptor.fontAttributes[@"NSCTFontUIUsageAttribute"] isEqualToString:@"CTFontEmphasizedUsage"]) {
fontName = FOBoldFontName;
}
else if ([descriptor.fontAttributes[@"NSCTFontUIUsageAttribute"] isEqualToString:@"CTFontObliqueUsage"]) {
fontName = FOItalicFontName;
}
else {
fontName = descriptor.fontAttributes[@"NSFontNameAttribute"];
}
return [UIFont fontWithName:fontName size:descriptor.pointSize];
}
self = [self initCustomWithCoder:aDecoder];
return self;
}
+ (void)load
{
[self replaceClassSelector:@selector(systemFontOfSize:) withSelector:@selector(regularFontWithSize:)];
[self replaceClassSelector:@selector(boldSystemFontOfSize:) withSelector:@selector(boldFontWithSize:)];
[self replaceClassSelector:@selector(italicSystemFontOfSize:) withSelector:@selector(italicFontOfSize:)];
[self replaceInstanceSelector:@selector(initWithCoder:) withSelector:@selector(initCustomWithCoder:)];
}
#pragma clang diagnostic pop
@end